A starter example tutorial for connecting ExpressJs to a Mongoose database hosted on MongoDB.
Prerequisites
Here's what you will need in order to get started:
- Setup a MongoDB account to host your database.
- We will continue on the simple starter Vue3/ExpressJS app from our prior post.
Getting Started
First, let's clone our prior Vue3/ExpressJS app and rename it. Run the following:
git clone git@github.com:Initial-Apps/vue3-express.git vue3-express-mongo
Now, let's move into our app and install our modules on our server
:
cd vue3-express-mongo/server
npm install
Next, let's start the server to make sure all is working. Run the following:
node server.js
Setting up MongoDB
Login to your MongoDB account: https://cloud.mongodb.com/
Select New Project
and name your project. You can add members if you like. Then click Create Project
.
Next, continue to Build a Database
.
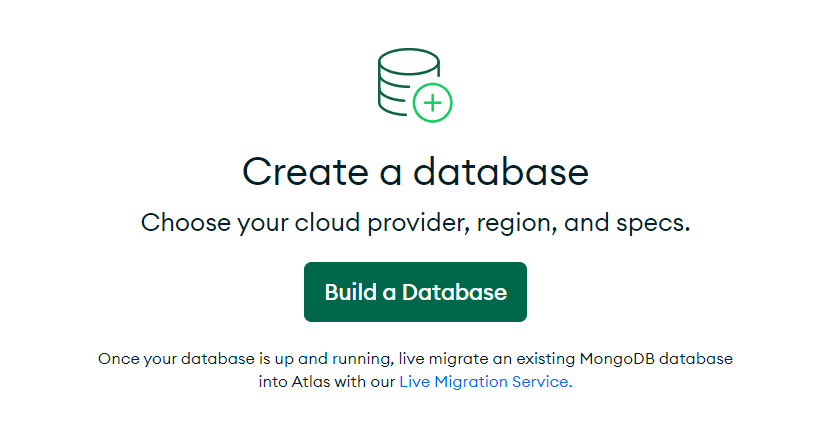
Select whatever plan you like and click create
.
Next, create a username and password for your database, and be sure to save this info.
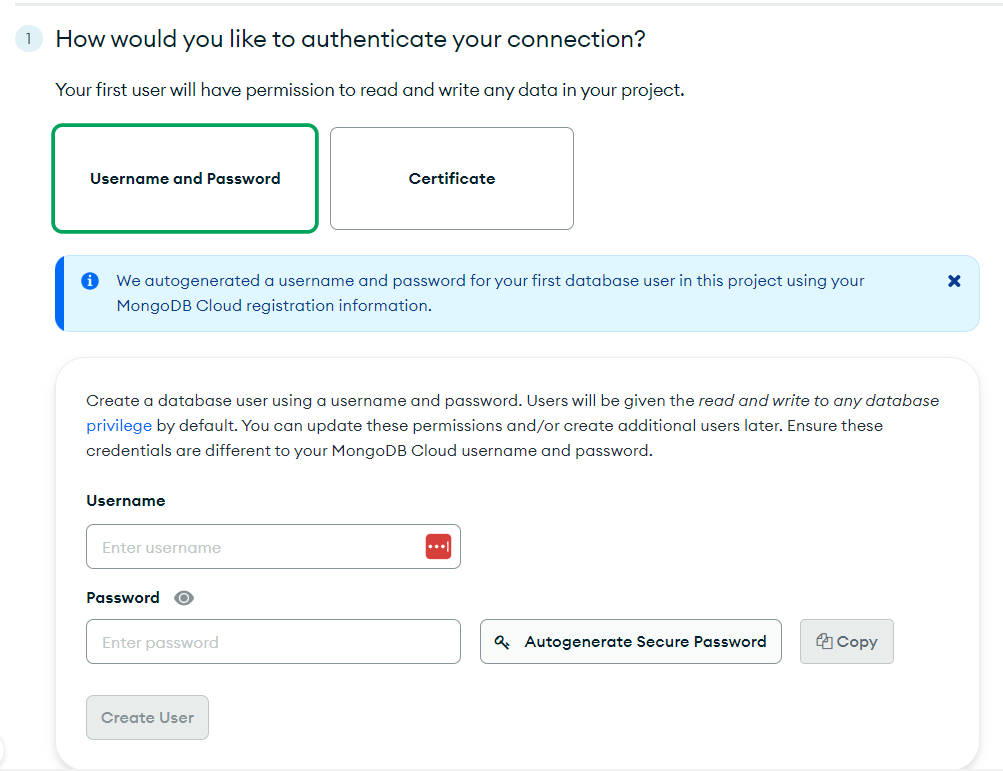
Then, select connect from My Local Environment
(see below):
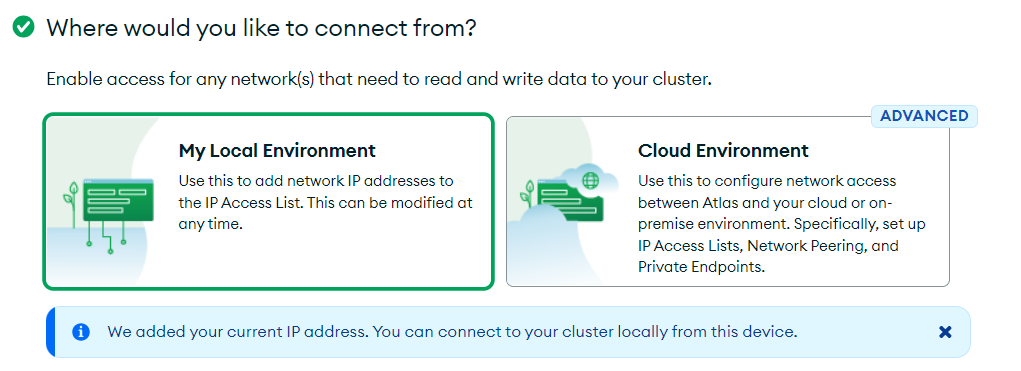
Finally, select Finish and Close
. Hooray, we've setup our database! 🤓
Connecting ExpressJs to our MongoDB
Now let's work on our server to connect ExpressJs to MongoDB. To do this, we'll work on our server middleware file server/server.js
. Let's open this file and get to work.
I'm adding the following code to server.js
just after defining our imported modules. I've added code comments as well.
require('dotenv').config(); // needed to read our environment variables
const mongoose = require('mongoose'); // We need the mongoose module
const connectString = process.env.MONGODB; // This holds our username and password, stored as a environment variable
mongoose.connect(connectString, { useNewUrlParser: true }).then(
() => {
console.log ('Succeeded connected to database');
},
err => {
console.log ('ERROR connecting to database: ' + err);
}
);
Here's what the above code does:
- Adds the
dotenv
module so that we can store our MongoDB username and password as an environment variable. This is preferred over placing this sensitive information directly in your code. - Adds the
mongoose
module for connecting to our MongoDB database - The
connectString
variable will hold our login credentials for MongoDB. We'll set this up next. - Finally, we add the connect script to connect to our DB.
Next, we have to setup our login and password for MongoDB as an environment variable. You do not want to ever share this file because it has sensitive login information. That said, I've created a example_env.txt
file in our github repo to provide template.
We make sure our environment credentials are hidden by opening your .gitignore
file, adding the following to the end of the file and save:
# env files
.env
Next, we'll get our connect string from MongoDB. You can find your connect string format on your MongoDB dashboard. Click connect
and then connect by Drivers.
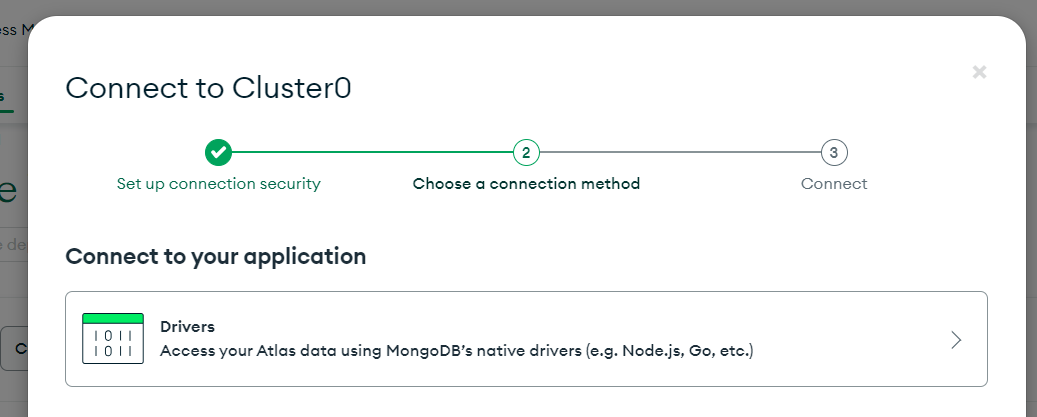
We are going to add our key using mongoose
so we only need to pay attention to the Driver and the Connection String.
Select Node
for the Driver like so:
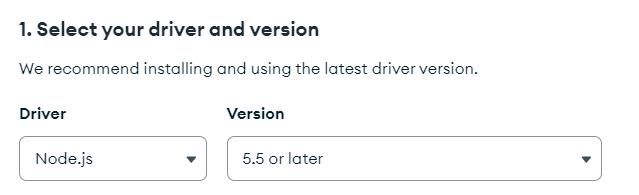
Then copy and paste your Connection String:
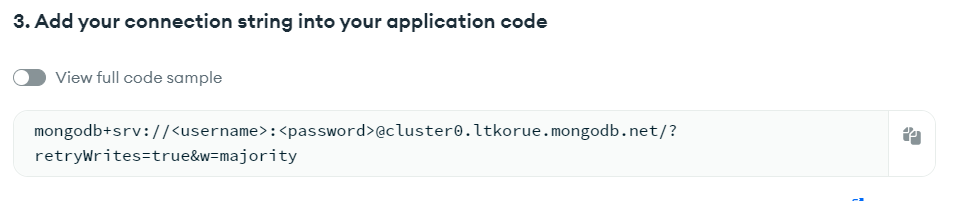
Now create a file named .env
and add your connect URL. The contents of the file should look something like this (replacing <username> and <password> with the MongoDB username and password we created in the last section). Save the file once this is added.
MONGODB=mongodb+srv://<username>:<password>@cluster0.ltkorue.mongodb.net/?retryWrites=true&w=majority
Then we install the modules we need for the connection. From the terminal window that we used to run our node server (the one that we ran node server.js
). First stop the server (hit ctrl+c
). Now install the modules:
npm install mongoose dotenv
Now we can restart our server by running:
node server.js
If all runs properly, you should see the following on your console:

Congrats as you are now connected to your database!
What's Next
Next, we need to start using your new database for various data! Want to see how we do that? Stay tuned or subscribe as we continue this app.